Your First SQL Query: Using SELECT to Retrieve Data from a Table
Writing your first SQL query can feel like a big leap, but trust me, it’s much simpler than it seems once you get used to writing SQL queries, and once you remember the syntax. The task you will do most often in SQL is retrieving data from a database, and you will SELECT statements for that. In this post, I’ll walk you through writing your first SQL query, and show you some very useful tricks like column aliasing and using DISTINCT to remove duplicates.
What is SQL SELECT Statement?
The SQL SELECT statement is the most important SQL query you will learn. It lets you retrieve data from one or more tables in a database. Whether you’re pulling a few specific columns or the entire table, or even multiple tables, SELECT is the command you will use.
Here’s what the basic SELECT statement looks like:
SELECT column_name FROM table_name;
It has the following elements:
- SELECT: This tells SQL you want to pull data from the database.
- column_name: These are the columns (fields) you want to retrieve from the database.
- FROM: This tells SQL that you want to select the data from some specific table.
- table_name: This is the name of the table holding your data.
Example:
If you open our Northwind database with SQL Server Management Studio, you will notice that there is a table called Employees:
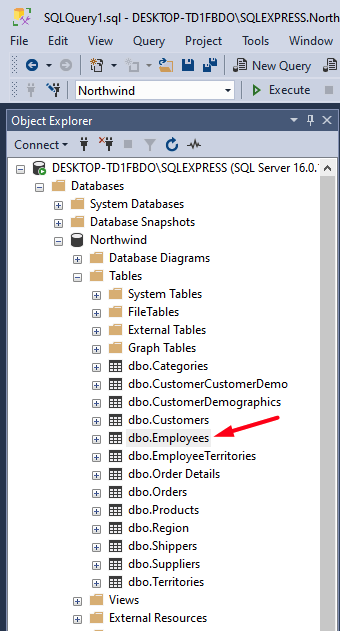
If you want to grab all the data from that table, you should click on New Query and write the following in it:
SELECT * FROM Employees;
It will look like this:
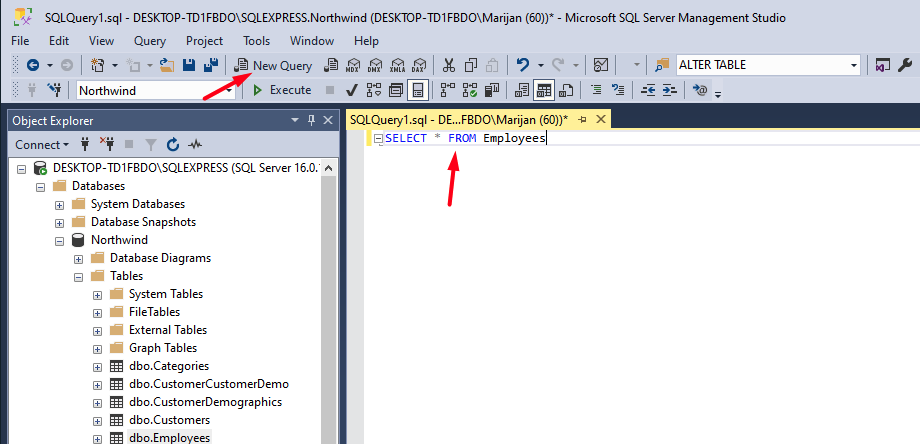
Now, when you click on Execute, you will see the results of your query:
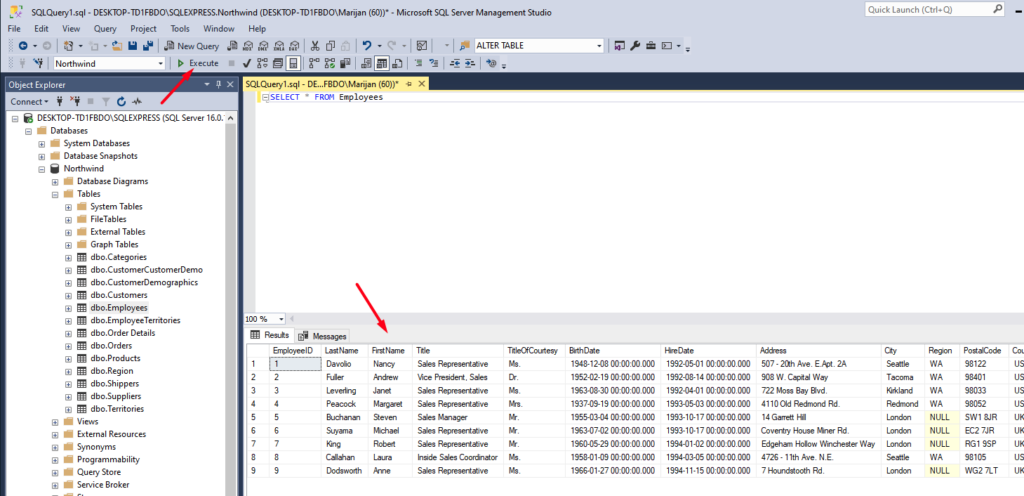
The asterisk *
is a character that tells SQL to select all the columns from the Employees table. This can be really helpful when you start writing your query, but it is usually better to select the columns you really need:
SELECT FirstName, LastName, Title FROM Employees
This version of the query retrieves just the First name, Last name, and Title columns. It’s cleaner and makes the result set more focused.
Here is what it looks like in SSMS, once we execute the statement:
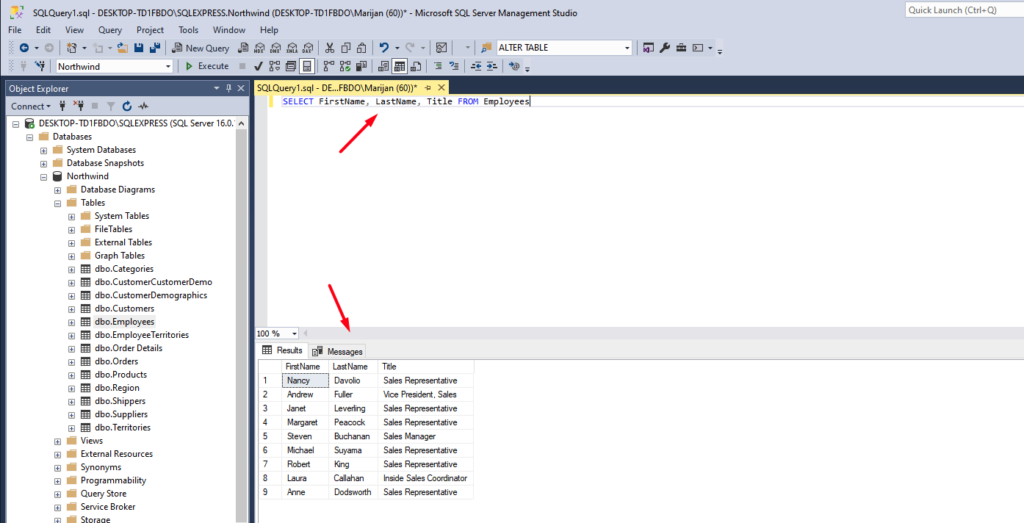
Aliasing Columns for Clarity
Sometimes, the names of columns in your database are not clear enough. By using the AS keyword, you can rename (or alias) columns to make the results easy to read.
Example:
Let’s use the Products table for this example. If you want to rename ProductID and SupplierID columns to show up as “Item Number” and “Vendor Number” in your results, you’d write something like this:
SELECT ProductID AS "Item Number"
,ProductName
,SupplierID AS "Vendor Number"
,CategoryID
,QuantityPerUnit
,UnitPrice
,UnitsInStock
,UnitsOnOrder
,ReorderLevel
,Discontinued
FROM Products
Here is what it looks like in SSMS:
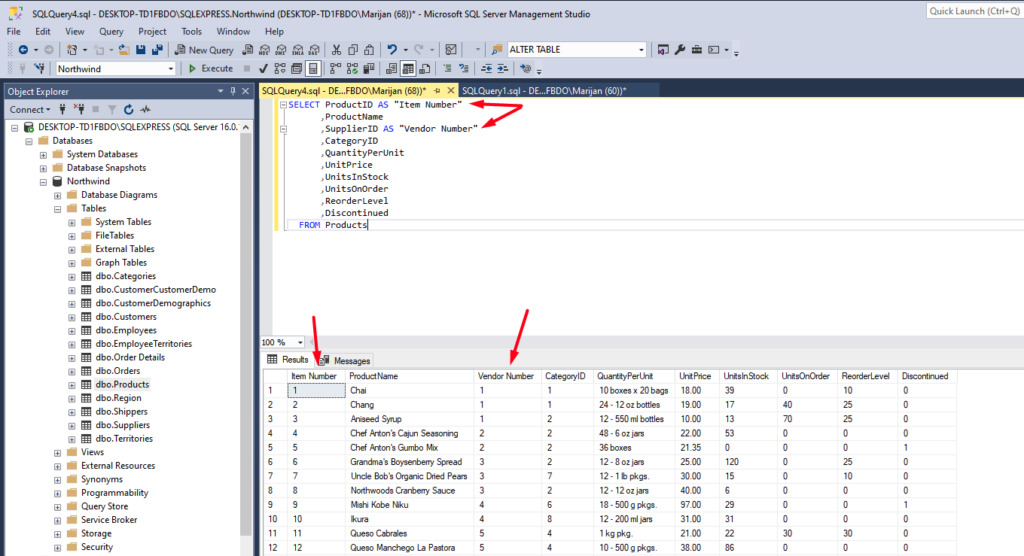
In this example, ProductID becomes “Item Number”, and SupplierID becomes “Vendor Number” in the output. This small change makes the results clearer, especially when you’re sharing the data with someone else.
Please keep in mind that this doesn’t rename the column in the table itself. It simply shows a new column name in the output.
Removing Duplicate Results with DISTINCT
Another useful statement you’ll come across when getting the data from the database is the SELECT DISTINCT statement. Very often, the queries can return duplicate rows, but you may only want unique values of a specific field. This is where you can use DISTINCT.
Example:
In our example, we have the table called Order. Write your first SQL query to get all columns and all records from the database. Here is what the query should look like:
SELECT * FROM Orders
When you run the SQL query, try scrolling down in the list of query results. You will notice that there are some duplicate Customer IDs:
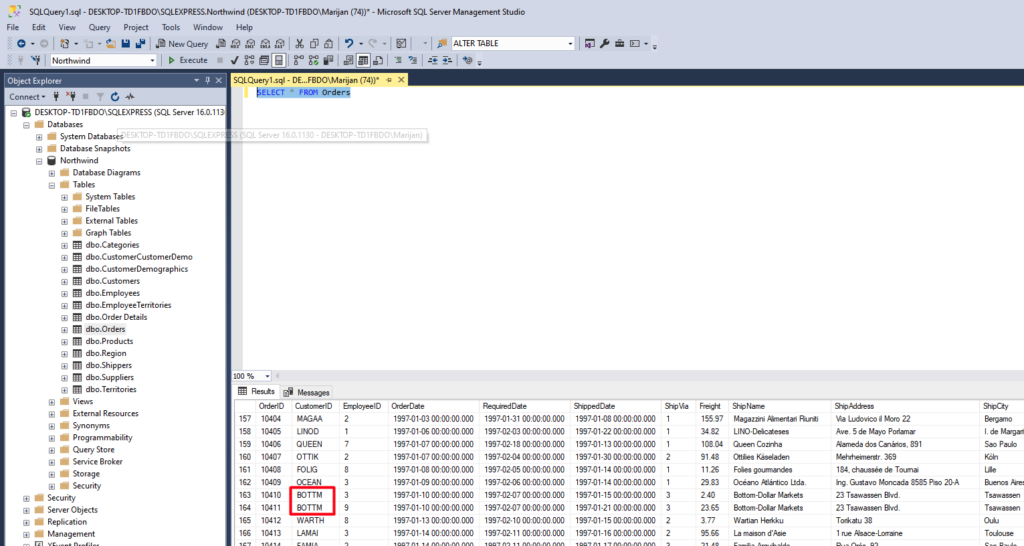
This absolutely makes sense because each customer can have multiple orders. If you would like to see only unique Customer ID values, you can use the following query:
SELECT DISTINCT CustomerID
FROM Orders;
Execute the query, and the results table will not contain any duplicate Customer IDs:
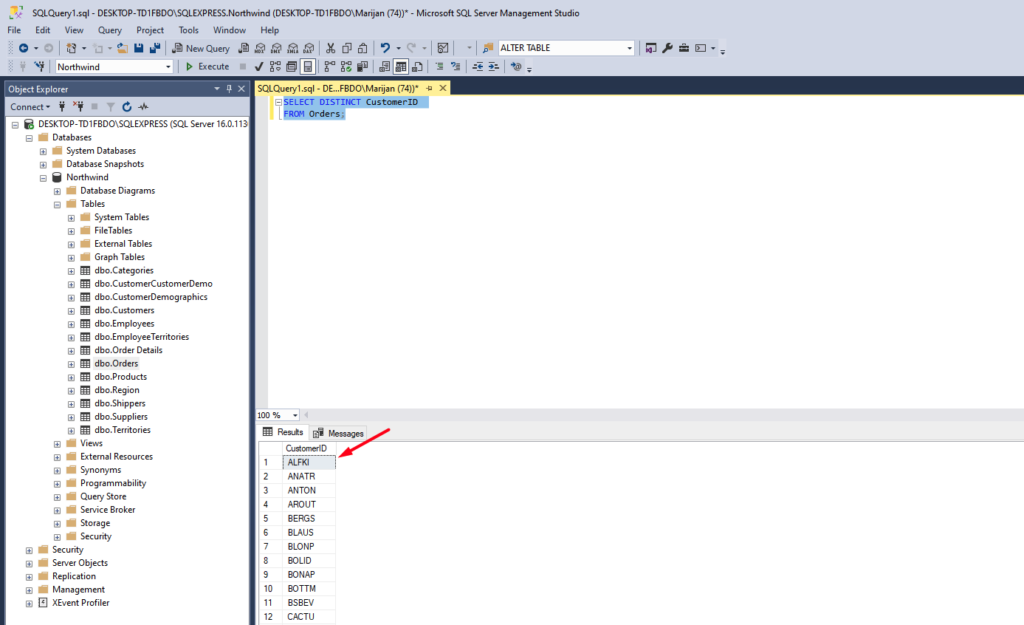
Using DISTINCT with Multiple Columns:
You can also apply the DISTINCT statement to multiple columns. For example, if you want to find unique combinations of customers and employees from which they ordered, you could write:
SELECT DISTINCT CustomerID, EmployeeID
FROM Orders;
This will prevent duplicate combinations of CustomerID and EmployeeID:
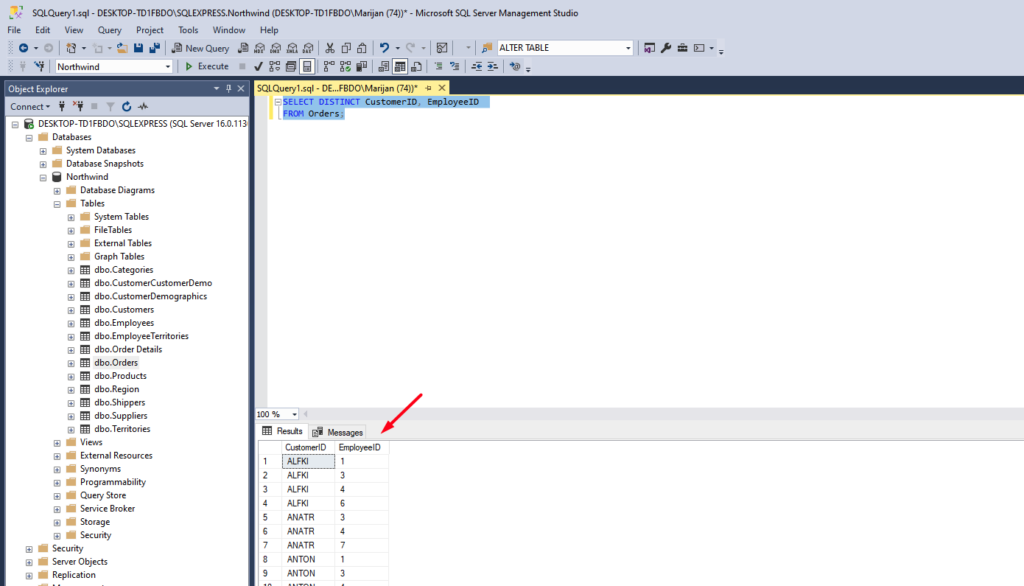
Wrapping Up
Writing your first SQL query is a key milestone when learning SQL, and mastering the SELECT statement is the foundation for everything that follows. From retrieving specific columns to using DISTINCT to remove duplicates, these are essential techniques you will use all the time.
If you haven’t already, try running some of these queries to get a feel for how they work. The more you practice, the more confident you’ll get. And once you’ve got the hang of it, you’ll be ready to dive into more advanced queries and really start exploring what SQL can do!