How to Do INNER JOIN in SQL?
If you’re working with SQL, understanding how to do INNER JOIN in SQL is essential. INNER JOIN is one of the most commonly used joins in SQL, allowing you to combine data from multiple tables based on a related column. In this guide, I’ll walk you through what INNER JOIN is, how it works, and provide practical examples to help you use it effectively.
What Is INNER JOIN in SQL?
An INNER JOIN retrieves only the matching records between two tables based on a specified condition. If a record doesn’t have a match in both tables, it won’t be included in the result set.
Here’s the basic syntax of an INNER JOIN:
SELECT table1.column1, table1.column2, table2.column1
FROM table1
INNER JOIN table2 ON table1.common_column = table2.common_column;
How to Do INNER JOIN in SQL?
Let’s say you have two tables:
- Customers (CustomerID, CompanyName, ContactName, Phone…)
- Orders (OrderID, CustomerID, OrderDate…)
To retrieve a list of customers along with their orders, you would use INNER JOIN like this:
SELECT cust.CustomerID, cust.CompanyName, cust.ContactName, cust.Phone, ord.OrderID, ord.OrderDate
FROM Customers AS cust
INNER JOIN Orders AS ord ON cust.CustomerID = ord.CustomerID
Here are the results in SQL Server Management Studio:
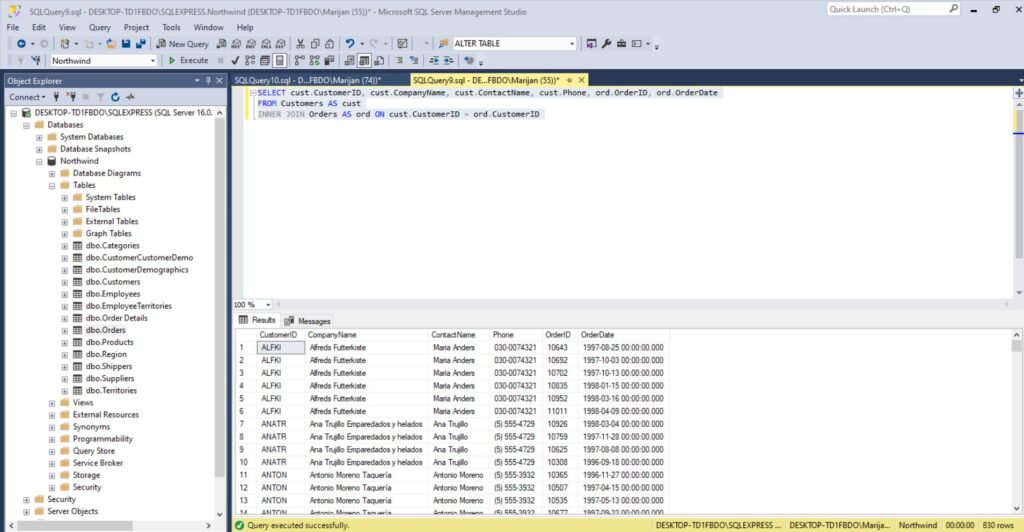
Did you notice how I’m using aliasing to keep things simple and differentiate from which tables I get each value?

This query joins the Customers and Orders tables based on the customer_id column, displaying only those customers who have placed orders.
How to Make INNER JOIN in SQL with Multiple Tables
You can also perform INNER JOIN with more than two tables. For example, if you have an additional Order Details table, you can join all three tables like this:
SELECT cust.CustomerID, cust.CompanyName, cust.ContactName, cust.Phone, ord.OrderID, ord.OrderDate, orddet.ProductID, orddet.Quantity, orddet.UnitPrice
FROM Customers AS cust
INNER JOIN Orders AS ord ON cust.CustomerID = ord.CustomerID
INNER JOIN [Order Details] AS orddet ON ord.OrderID = orddet.OrderID
You can notice that now we can display values from all of these tables:
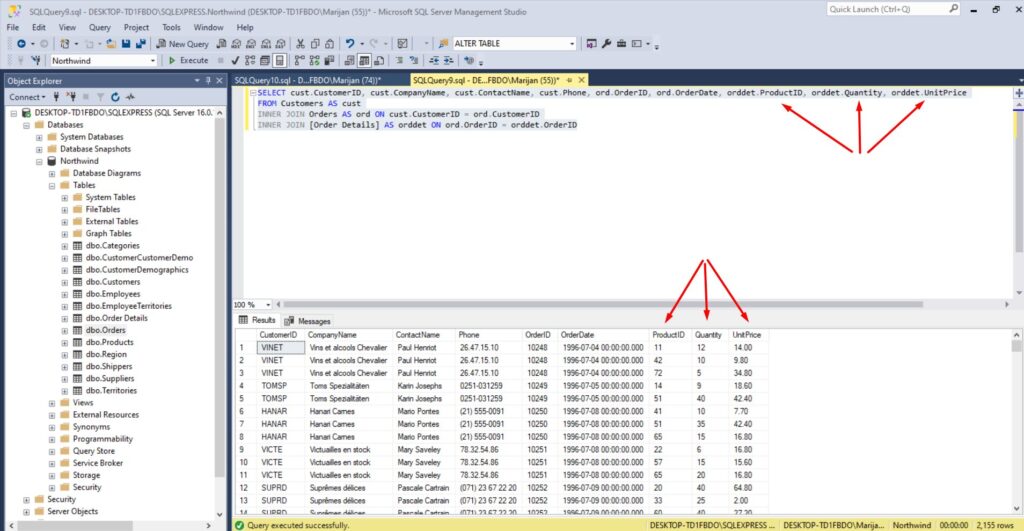
This method ensures you retrieve only the records that have matching entries in all three tables.
Why Use INNER JOIN Instead of Other Joins?
- Precise Matching: Only retrieves rows that exist in both tables.
- Faster Performance: Compared to OUTER JOINs, INNER JOINs are usually more efficient.
- Clean Results: Avoids NULL values that can appear in LEFT or RIGHT JOINs.
Conclusion
Now that you know how to do INNER JOIN in SQL, you can confidently join tables and retrieve related data. Whether working with two tables or multiple, INNER JOIN ensures your queries return only matching records, making your SQL queries more efficient. Try applying INNER JOIN in your database and see how it enhances your data retrieval!
Have fun!
PS. Make sure you check one of my previous blog posts on How to Use GROUP BY and HAVING Clause in SQL.
If you would like to learn more about SQL queries, make sure you buy this book: T-SQL Fundamentals (Developer Reference)