How to Select Specific Columns in SQL?
When working with databases, you rarely need to retrieve every column from a table. Instead, you often select specific columns to get only the data you need. Learning how to select specific columns in SQL is essential for writing efficient queries, improving readability, and reducing unnecessary processing time.
In this blog post, I’ll explain how to retrieve specific columns from a table, how to use aliases to make your queries more readable, and how to get column names as rows when needed. Let’s dive in!
How to Select Specific Columns in SQL
To retrieve specific columns from a table, use the SELECT
statement followed by the column names you want to include in your result set. This approach reduces the amount of data returned by your query, making it more efficient.
Here’s the basic syntax:
SELECT column1, column2
FROM table_name
For example, if you have a Employees
table and want only the employee’s ID, name and country, you’d write:
SELECT EmployeeID,LastName,FirstName,Country
FROM Employees
This query retrieves only the Employee ID
, Last Name
, First Name
and Country
columns, ignoring all others.
Here is what it looks like in SQL Server Management Studio:
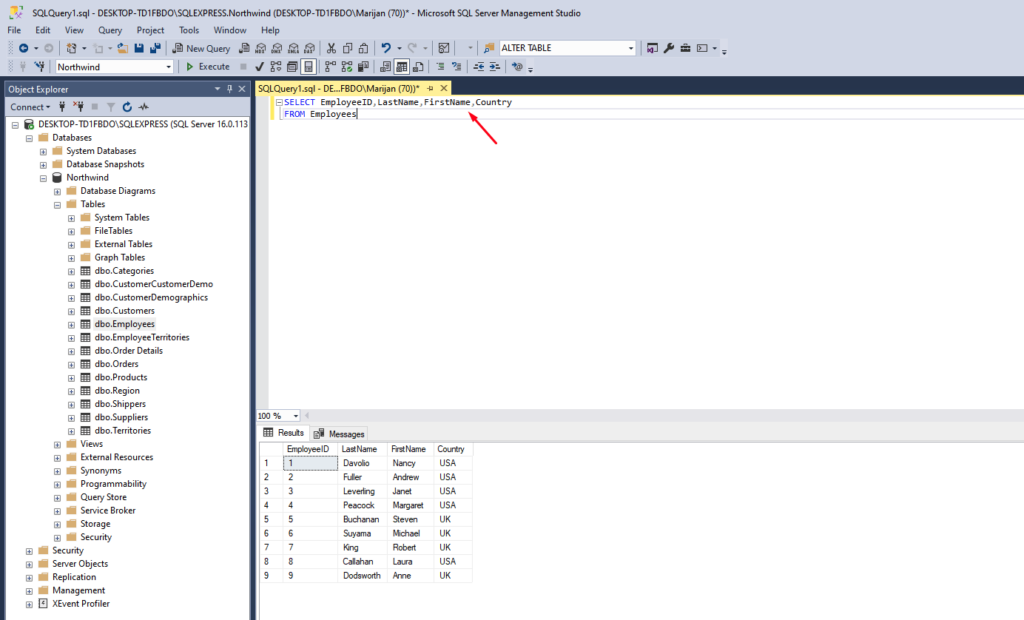
Using Aliases for Readability
Aliases allow you to rename columns or tables temporarily for the duration of a query. This is particularly useful when:
- You want to simplify long or complex column names.
- You need to create a more user-friendly output.
- You’re combining multiple tables with similar column names.
To use an alias, add the AS
keyword followed by the new name.
For example, suppose you want to rename EmployeeID
to EmployeeNumber
, City
to CityOfResidence
and Country
to CountryOfResidence
:
SELECT
EmployeeID AS EmployeeNumber
,City AS CityOfResidence
,Country AS CountryOfResidence
FROM Employees
Aliases make your results easier to read, especially in reports or user-facing outputs. You can also notice that I write each field in a new line. It simply makes it easier to read your SQL query.
Here is what it looks like in SSMS:
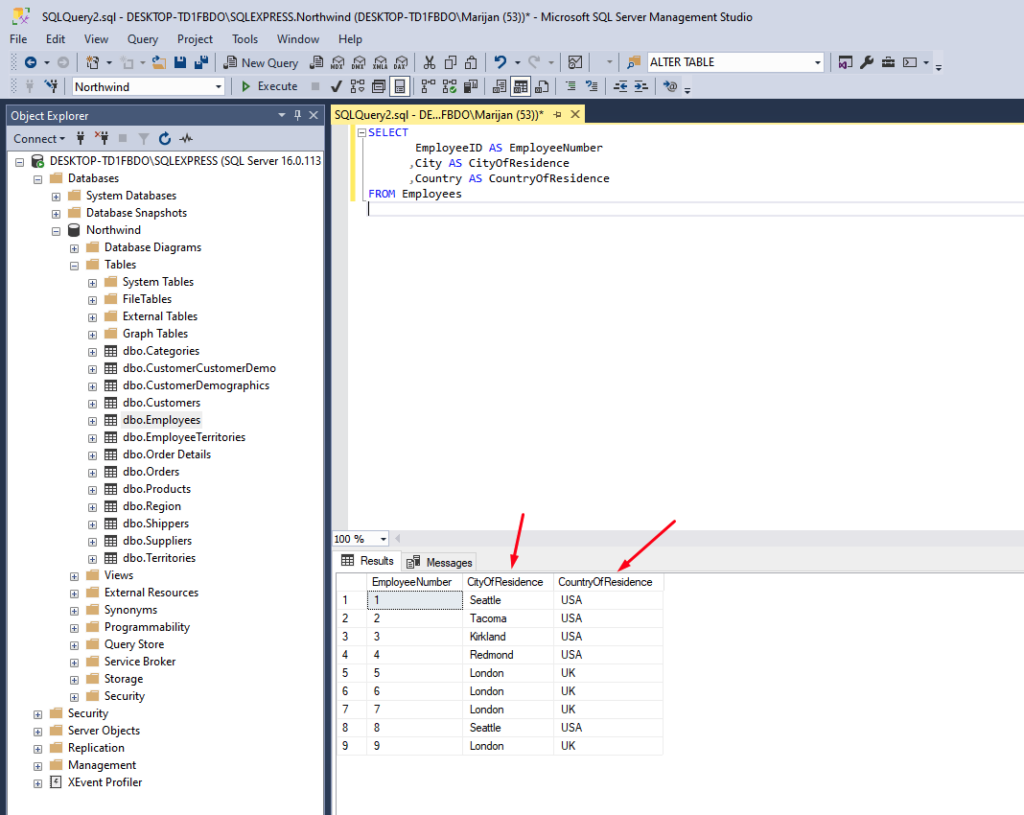
Note: While AS
is optional in most SQL dialects, using it explicitly improves clarity. But you can also write it like this, and you will get the same results:
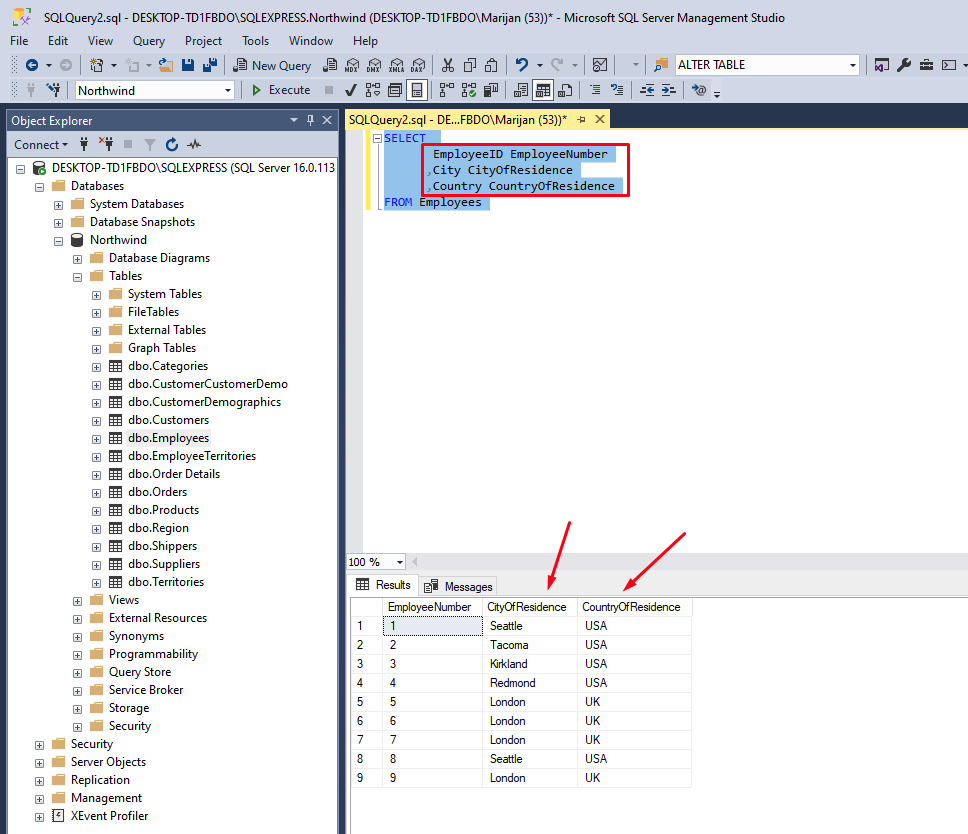
Select Columns from SQL Table
Sometimes, you need to list all the column names of a table as rows, such as when documenting your database structure or debugging. SQL Server doesn’t have a direct query for this, but you can use the system catalog view INFORMATION_SCHEMA.COLUMNS
.
Here’s how you can select columns from the SQL table as rows:
SELECT COLUMN_NAME
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = 'YourTableName'
Replace 'YourTableName'
with the name of your table. For example, to get column names for a table named Employees
:
SELECT COLUMN_NAME
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = 'Employees'
This query returns a list of column names in the Employees
table, which can be especially helpful during development or troubleshooting.
Here is what it should look like on your screen:
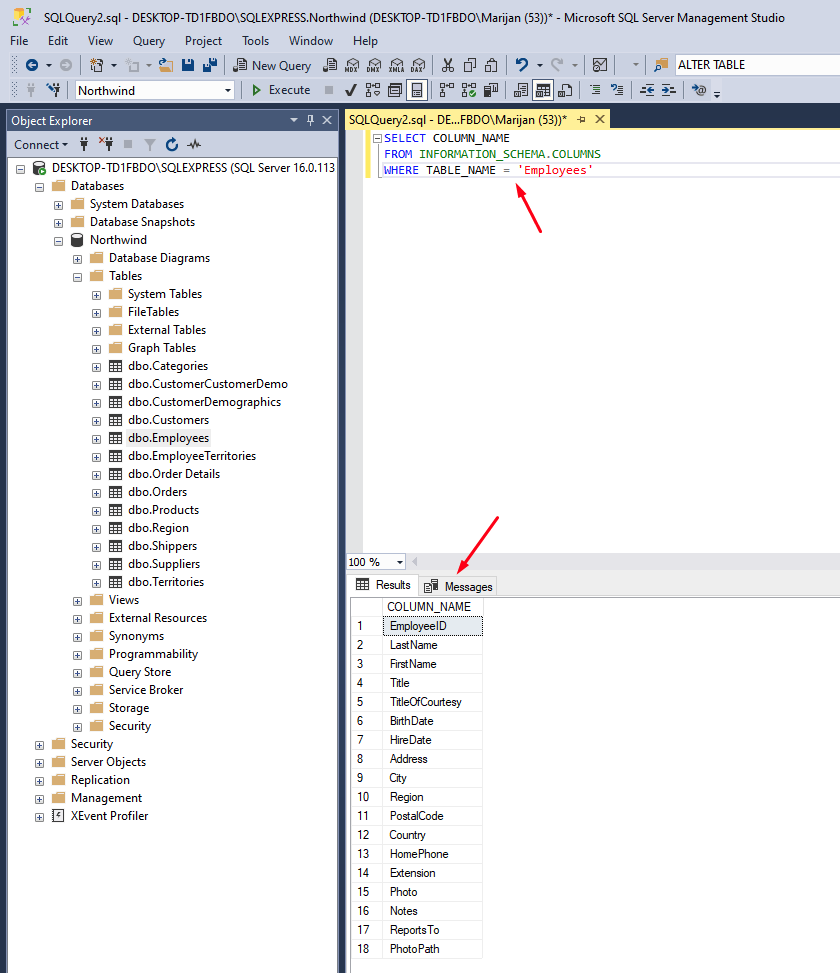
Combining Aliases with Column Selection
To take it one step further, you can combine aliasing with retrieving column names dynamically. For instance, you might want to retrieve column names alongside their data types:
SELECT COLUMN_NAME AS ColumnName, DATA_TYPE AS DataType
FROM INFORMATION_SCHEMA.COLUMNS
WHERE TABLE_NAME = 'Employees'
This query provides a detailed view of the table’s structure, including the column names and their respective data types.
Conclusion
Knowing how to select specific columns in SQL is a fundamental skill that enables you to focus on relevant data, improve query efficiency, and create clean and readable outputs. By using aliases, you can make your queries more intuitive, and by retrieving column names as rows, you can better understand your table structure when needed.
If you’re working on a new SQL project or testing database queries, start by selecting only the columns you need. It’s a simple but powerful practice that will save you time and resources.
Make sure you check my last post on how to Limit Results on SQL Server.
If you would like to learn more about SQL, check out this amazing book on Amazon.
What other SQL tips or challenges do you have? Share them in the comments below—I’d love to help!