Limit Results on SQL Server: Using the SQL TOP Statement
When working with large datasets in Microsoft SQL Server, you don’t always need the entire result set. Sometimes, you only want a part of the dataset. Whether you’re retrieving the top 5 customers, the top 10 highest-paid employees, or simply testing your queries, the SQL TOP statement could help you achieve this. Limiting results is especially helpful during query development, as it allows you to test and refine your queries without processing the entire dataset.
In this guide, I’ll show you how to limit results on SQL Server using the TOP statement and compare it with the LIMIT statement often used in other database systems.
What is the SQL TOP Statement?
The SQL TOP statement allows you to retrieve a specific number or percentage of rows from a query’s result set. It’s perfect for scenarios where you need only a portion of the data instead of everything in a table.
Here’s the basic syntax:
SELECT TOP (number) column1, column2
FROM table_name
[ORDER BY column_name ASC|DESC]
- number: The number of rows you want to retrieve
- ORDER BY: While optional, it’s crucial for determining which rows to include in the “top” results. Without it, the order of returned rows may vary.
Limiting Results with a Fixed Number
To limit results to a specific number of rows, use the TOP keyword followed by the number of rows.
For example, to retrieve the top 10 products with highest Unit Price:
SELECT TOP (10)
ProductID
,ProductName
,UnitPrice
FROM Products
ORDER BY UnitPrice DESC
This query selects the 10 products with the highest prices, sorted in descending order.
Here is what it looks like in SQL:
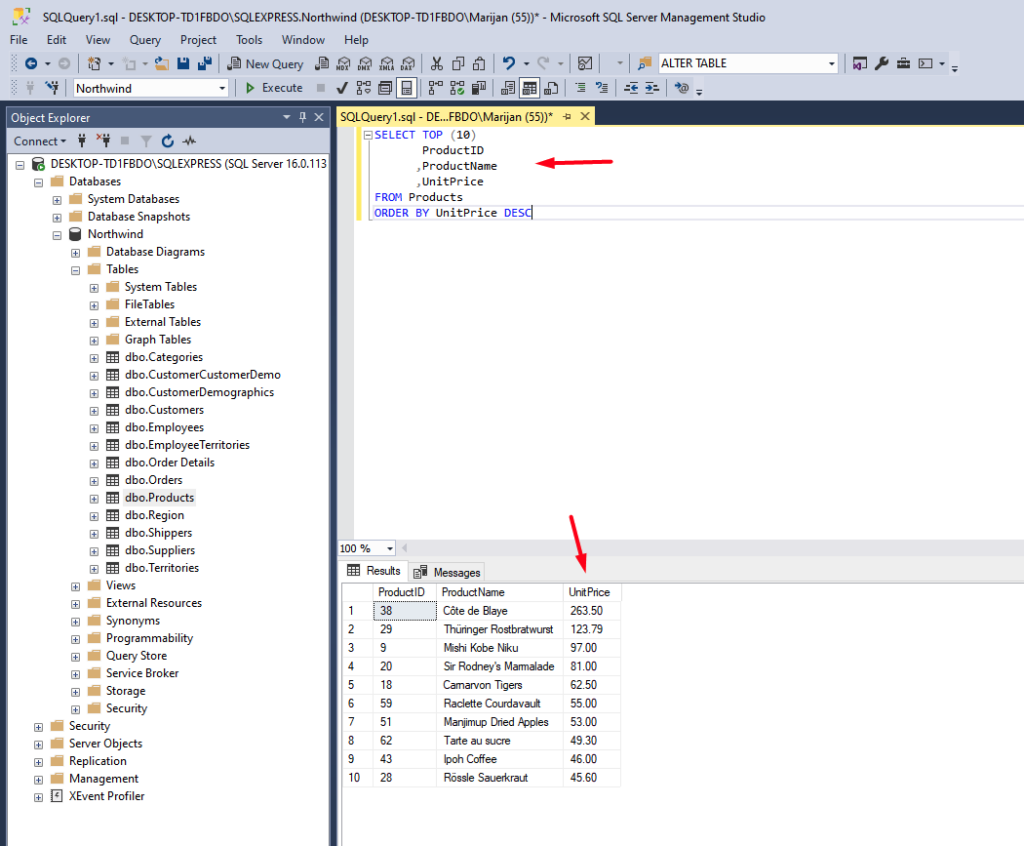
Limiting Results by Percentage
Instead of retrieving a fixed number of rows, you can use the PERCENT keyword to retrieve a proportional selection of rows from the result set.
For instance, to retrieve the top 20% of products with the highest Unit Price, you can write the following SQL query:
SELECT TOP (20) PERCENT
ProductID
,ProductName
,UnitPrice
FROM Products
ORDER BY UnitPrice DESC
This is useful for scenarios where the size of the dataset can vary, but you always want a proportional subset of results.
This is what it looks like in SSMS:
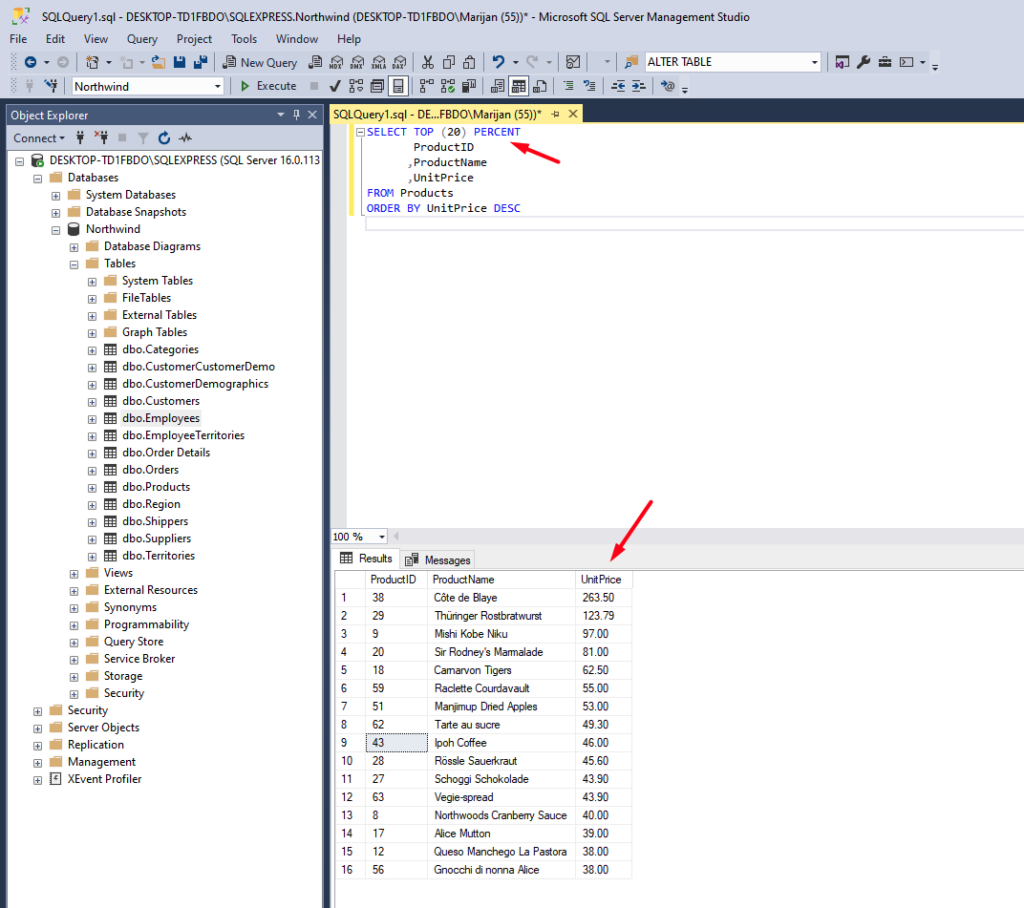
SQL TOP vs LIMIT
If you’ve worked with databases like MySQL or PostgreSQL, you might be familiar with the LIMIT keyword. While TOP is used in SQL Server, LIMIT is the equivalent in many other database systems.
Here’s a comparison to help you understand SQL TOP vs LIMIT:
Syntax:
In SQL Server:
SELECT TOP (5) column1, column2
FROM table_name
ORDER BY column_name
In MySQL/PostgreSQL:
SELECT column1, column2
FROM table_name
ORDER BY column_name
LIMIT 5
Flexibility:
TOP works with percentages (e.g., TOP (10) PERCENT), while LIMIT does not support this feature directly.
Placement:
TOP appears immediately after the SELECT keyword.
LIMIT is typically placed at the end of the query.
If you’re migrating queries between databases, understanding SQL TOP vs LIMIT can help you adapt your queries to the target database system.
Using TOP with Conditions
The TOP statement becomes even more powerful when combined with the WHERE clause to filter data before limiting rows.
For example, to retrieve the top 5 products with the highest Unit Price in Category ‘8’:
SELECT TOP (5)
ProductID
,ProductName
,UnitPrice
FROM Products
WHERE CategoryID = '8'
ORDER BY UnitPrice DESC
This query first filters products in Category ‘8’ and then limits the result to the top 5 with the highest price.
Here is what it should look like on your screen:
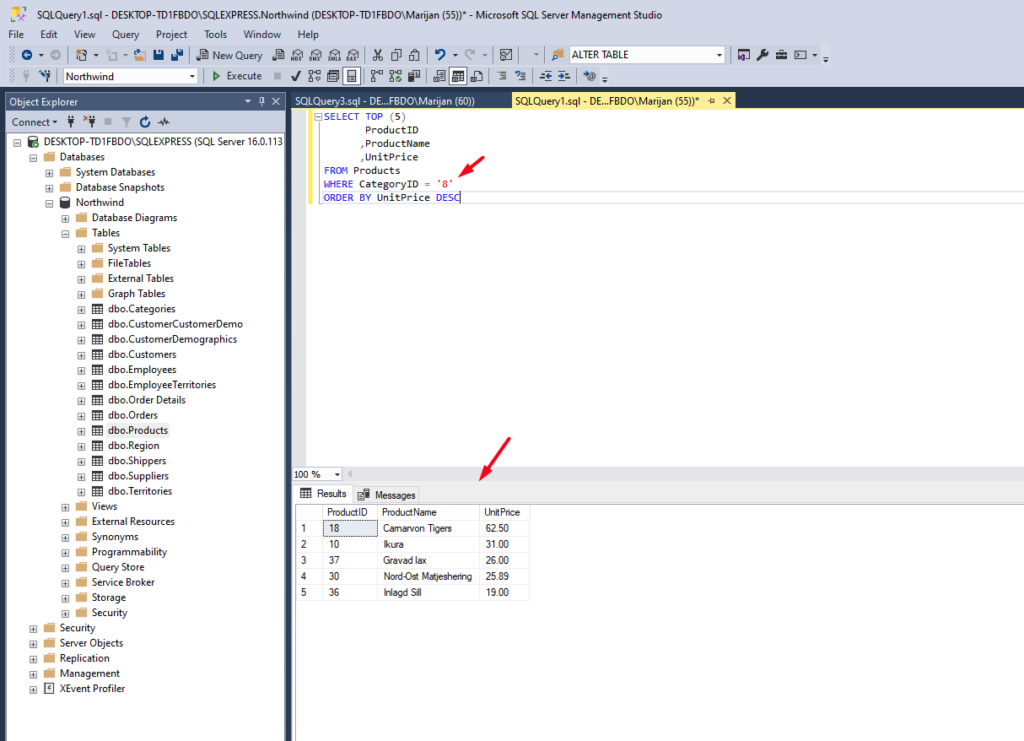
Conclusion
The SQL TOP statement is a very useful tool to limit results on SQL Server, making it ideal for everything from large dataset analysis to testing and debugging queries. Whether you’re working with a fixed number of rows, percentages, or filtered data, mastering the TOP statement is an essential skill for efficient querying.
For those familiar with other databases, knowing the difference between SQL TOP vs LIMIT will help you write queries that are optimized for SQL Server while easily transitioning to other systems when needed.
Start experimenting with the TOP statement today and see how it simplifies your workflows. Have questions or additional insights? Share them in the comments below!
Also, make sure you check my last post about SQL called Sorting Data in SQL: A Guide to the SQL ORDER BY Statement
If you would like to learn more about SQL, check out this amazing book on Amazon.