Mastering the SQL WHERE Clause: A Free 20-Minute Guide to Filtering Data
When working with SQL, one of the most fundamental things you’ll need to do is filter data from your database. Filtering allows you to retrieve just the records you need rather than going through all the data inside your table. In this post, I will dive into using the SQL WHERE clause to filter records based on specific conditions. Along the way, I’ll also show you some practical examples of how you can get the most out of the WHERE clause, including using it with multiple values, date ranges, and even a CASE statement.
What is the SQL WHERE Clause?
The SQL WHERE clause filters records based on one or more conditions. When you use SELECT to query data from a table, the WHERE clause allows you to specify which rows should be included in the results. Without it, you’d return all the data from the table, which often isn’t what we want.
The syntax for the SQL WHERE clause looks like this:
SELECT column1, column2
FROM table_name
WHERE condition;
For example, if you want to select all the employees from Employee table, you would run the following query:
SELECT *
FROM Employees
Here are the results without the WHERE clause:
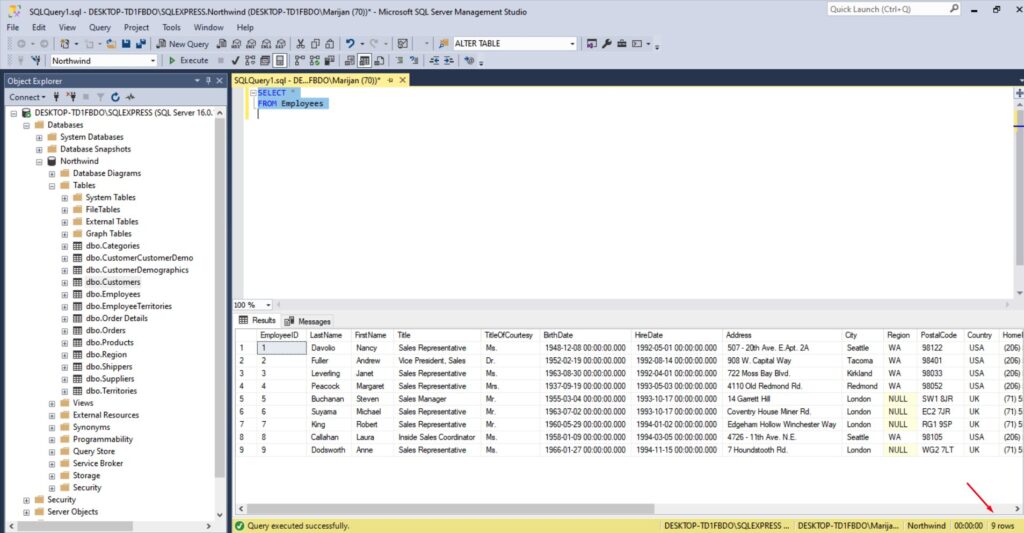
If you would like to filter all the employees living in Seattle, you need to run this query:
SELECT *
FROM Employees
WHERE City = 'Seattle'
After you run the query, you will get the following results (notice how you have only 2 rows now):
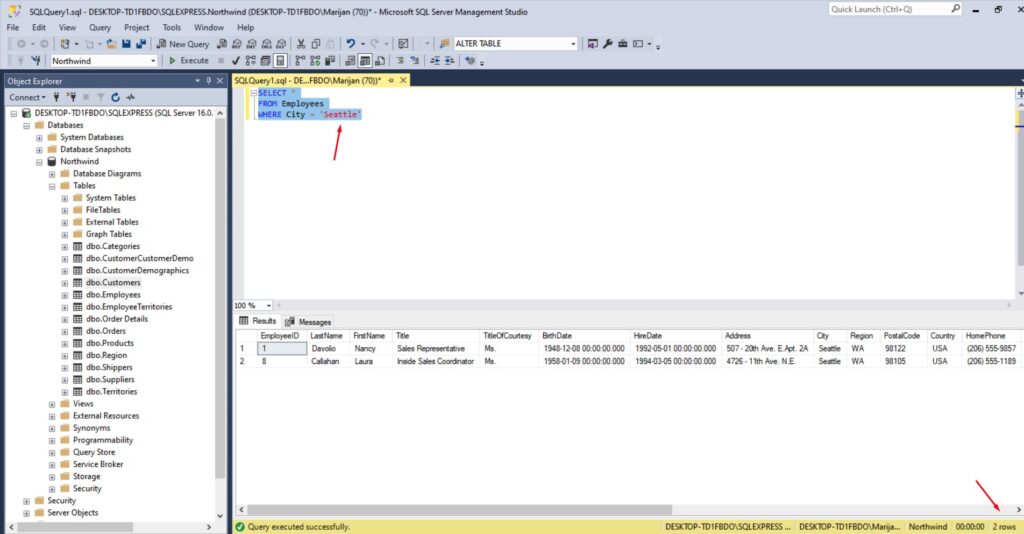
Now, let’s go deeper and explore how you can use the WHERE clause in more advanced ways.
SQL WHERE Clause with Multiple Values
One of the most common scenarios you’ll encounter is the need to filter records based on multiple values. For this, SQL provides the IN operator, which is perfect for handling multiple conditions within the WHERE clause. Let’s say you have a table with customer data and you only want to retrieve records for customers based in either Germany or Mexico.
Here’s how you would use the SQL WHERE clause with multiple values:
SELECT *
FROM Customers
WHERE Country IN ('Germany','Mexico')
In this case, the query will return all customers whose country is either ‘Germany’ or ‘Mexico’. This is much simpler than writing multiple OR conditions and keeps your code clean, but you can also write something like this, using OR operator:
SELECT *
FROM Customers
WHERE Country = 'Germany' OR Country = 'Mexico'
In both cases, you will get the following results:
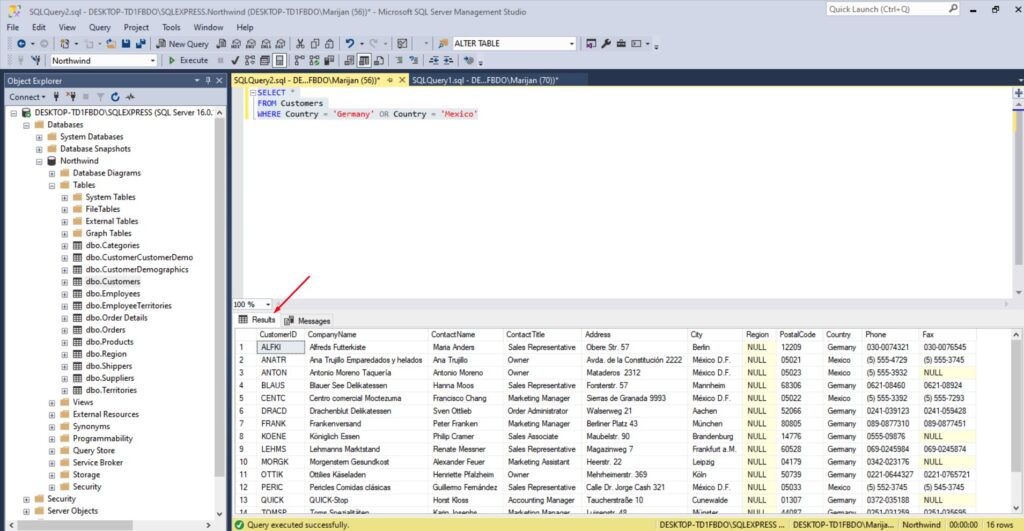
SQL WHERE Clause Between Two Dates
Another common use of the SQL WHERE clause is filtering data between two dates. Whether you’re working with orders, transactions, or any kind of time-sensitive data, you often need to retrieve records within a certain time frame.
For instance, if you want to pull all orders placed between January 1st, 2024, and December 31st, 2024, you’d use the BETWEEN … AND operator in the WHERE clause like this:
SELECT *
FROM Orders
WHERE OrderDate BETWEEN '1998-01-01' AND '1998-12-31'
The beauty of the BETWEEN … AND operator is that it includes both boundary values, meaning it will return records where the order date is exactly ‘2024-01-01’ or ‘2024-12-31’, as well as all dates in between.
Here is the result set you will see for the year 1998:
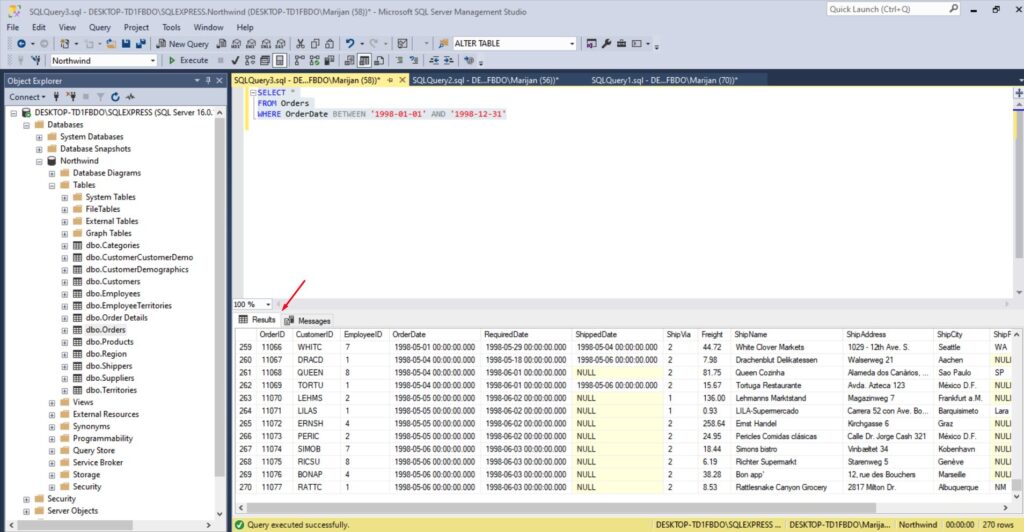
Filtering with Comparison Operators
Sometimes, filtering isn’t just about specific values or ranges. You might need to filter data based on a comparison. SQL provides several comparison operators like =, >, <, >=, <=, and <> (not equal).
For example, if you want to filter orders with a quantity greater than 10, you can write the query like this:
SELECT *
FROM [Order Details]
WHERE Quantity > 10
This query will return all order lines whose quantity exceeds 10. You can use any of the comparison operators depending on your needs.
SQL WHERE Clause with CASE Statement
In more complex scenarios, you might need to apply different conditions based on certain values in your data. That’s where the CASE statement comes in handy. You can use the CASE statement within the SQL WHERE clause to apply conditional logic to your queries.
While the CASE expression cannot be used in the WHERE clause as a direct condition, you can still use it within a CASE statement in combination with logical values. This approach can be a bit unconventional but looks something like this:
For instance, let’s say you have products from different categories, and you want to filter products based on their category and unit price. For the category ID = 2, you want to filter those with a unit price greater than 10, and for category ID = 6, those with a unit price above 50. Here’s how you can do that using a CASE statement:
SELECT *
FROM Products
WHERE
CASE
WHEN CategoryID = 2 AND UnitPrice > 10 THEN 1
WHEN CategoryID = 6 AND UnitPrice > 50 THEN 1
ELSE 0
END = 1;
In this query:
- The CASE expression evaluates the specified conditions.
- If CategoryID is 2 and UnitPrice > 10, or if CategoryID is 6 and UnitPrice > 50, the CASE expression returns 1.
- Otherwise, it returns 0.
- The WHERE clause then checks if the result is 1 (meaning the row meets one of the specified conditions).
Here is what the result set looks like:
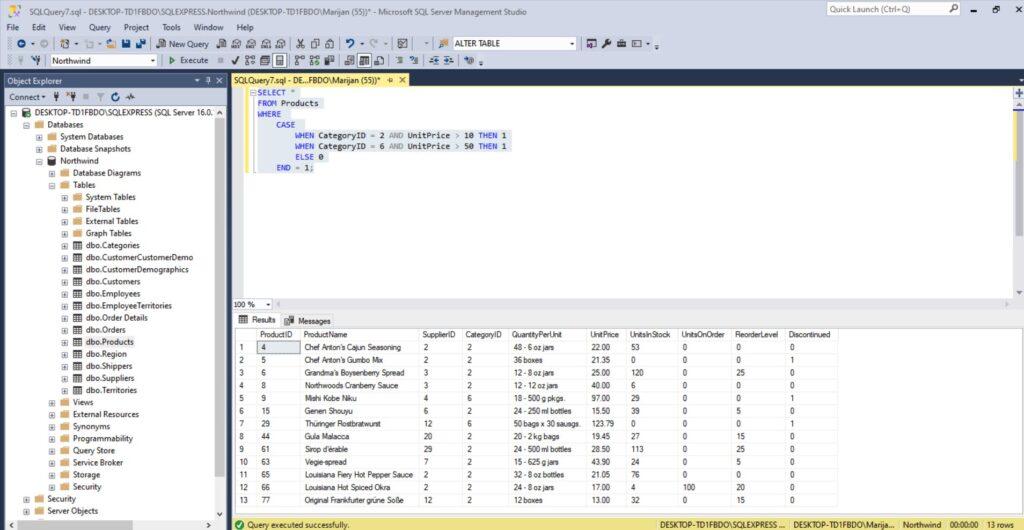
This approach allows you to write more dynamic SQL queries that change their filtering logic based on the data itself. While the CASE statement might not be something you use every day, it’s incredibly powerful when you need it.
Filtering Null Values
Another important feature of the SQL WHERE clause is handling NULL values. Sometimes, data might be incomplete, and fields may contain NULL values, representing missing or undefined information. SQL provides two special operators for this: IS NULL and IS NOT NULL.
For example, if you want to retrieve all customer where the region is not set, you can use this query:
SELECT *
FROM Customers
WHERE Region IS NULL
This query will return all orders where the DeliveryDate field is empty.
Using AND and OR for Multiple Conditions
Finally, here is one more example on how to combine multiple conditions in the WHERE clause using AND and OR. These operators allow you to filter data based on several conditions at once.
For example, let’s say you want to retrieve products where supplier ID = 2, and with a unit price greater than 20. You would write the query like this:
SELECT *
FROM Products
WHERE SupplierID = 2 AND UnitPrice > 20
On the other hand, if you want to filter records that meet either of two conditions, you can use OR. For instance, you might want to retrieve orders that ship to either France or Brazil:
SELECT *
FROM Orders
WHERE ShipCountry = 'France' OR ShipCountry = 'Brazil'
Quick Tip: Using WHERE 1=1 in SQL
If you’ve ever come across the WHERE 1=1 clause in SQL queries and wondered why it’s used, here’s a quick tip on its purpose.
In SQL, WHERE 1=1 is a dummy condition that always evaluates to TRUE. While it doesn’t filter any rows by itself, it’s often used as a placeholder when dynamically building queries in code. The main benefit is that it simplifies adding additional WHERE conditions, especially in complex queries or when using conditional logic in scripts.
For example, we want to filter orders that ship to either France or Brazil, and where ShipRegion is not null. We would run the following query:
SELECT *
FROM Orders
WHERE 1 = 1
AND (ShipCountry = 'France' OR ShipCountry = 'Brazil')
AND ShipRegion IS NOT NULL
Now, if we want to add the additional condition, we would just add the new line at the end of our query:
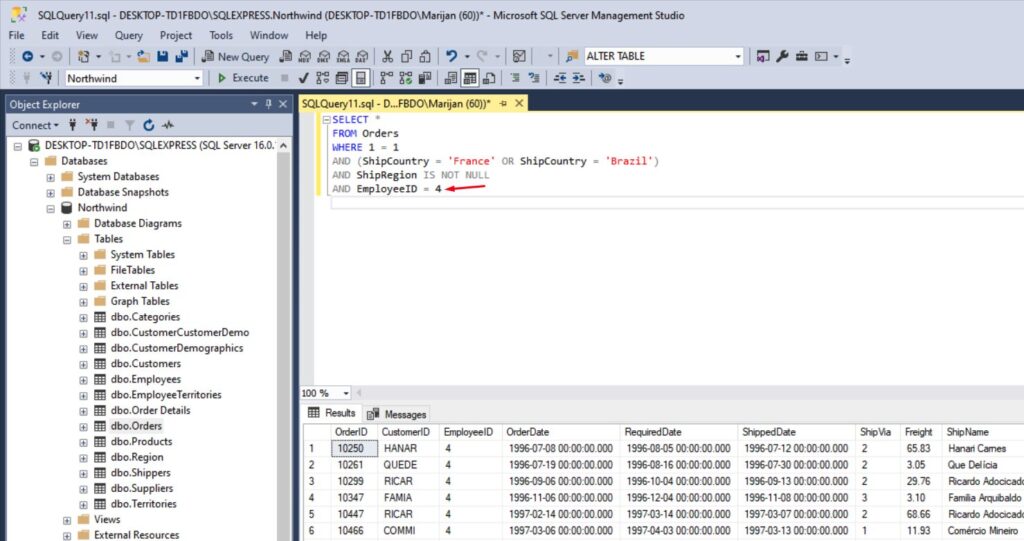
By starting with WHERE 1=1, you can easily append multiple conditions without worrying about whether the query already has an existing WHERE clause. It ensures that all conditions after 1=1 can be added with an AND without having to check for query structure.
This little trick helps avoid syntax issues when dynamically building queries and keeps your SQL clean and flexible!
Conclusion
The SQL WHERE clause is one of the most versatile and useful tools for filtering data in SQL queries. Whether you’re using the WHERE clause with multiple values, filtering between two dates, or using a CASE statement for more advanced logic, it gives you the power to retrieve only the data you need.
If you’re just getting started, practice using the SQL WHERE clause with various conditions to see how flexible it can be. In my next post, I’ll dive deeper into using aggregate functions and filtering with the HAVING clause, so stay tuned!
If you missed it, make sure you read my previous post on SQL queries called Your First SQL Query: Using SELECT to Retrieve Data from a Table.
If you would like to learn more about SQL, check out this amazing book on Amazon.